Table Of Content
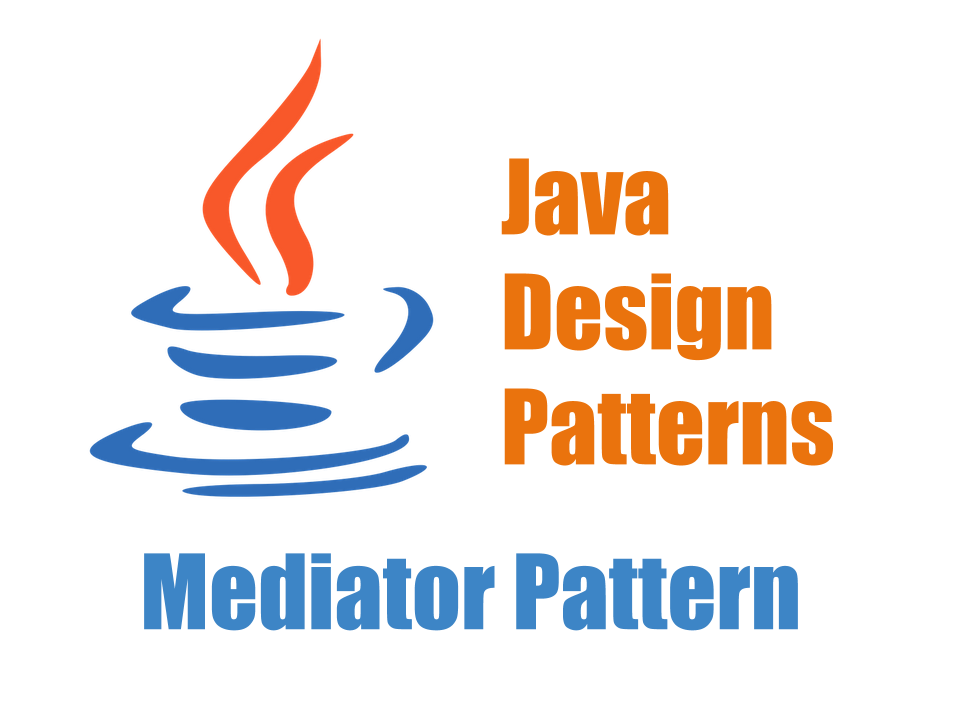
The system objects that communicate each other are called Colleagues. Usually we have an interface or abstract class that provides the contract for communication and then we have concrete implementation of mediators. For our example, we will try to implement a chat application where users can do group chat. Every user will be identified by it’s name and they can send and receive messages.
The Mediator Pattern in the Spring Framework
Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and it lets us vary their interaction independently. Design patterns are used to solve common design problems and reduce the complexities in our code. The mediator pattern is a behavioral design pattern that promotes loose coupling between objects and helps to organize the code for inter-object communications. Mediator is a behavioral design pattern that reduces coupling between components of a program by making them communicate indirectly, through a special mediator object.
R as in Ridiculous .. The first impression for a software engineer
The Mediator Pattern, which is similar to the Command, Chain of Responsibility, and Iterator patterns, are part of the Behavioral pattern family of the Gang of Four design patterns. Behavioral patterns address responsibilities of objects in an application and how they communicate between them. The Mediator pattern allows the loose coupling between set of objects in an application by handling the interactions between the objects. Note that the mediator design pattern differs from the facade design pattern. The mediator pattern facilitates how a set of objects interact, while the facade pattern simply provides a unified interface to a set of interfaces in the application.
Mediator Pattern
Also, in both the classes we implemented the overridden attack() and stopAttack() methods declared in the ArmedUnit interface. In the attack() method, we communicated with the mediator by calling the canAttack() method. If the method returns true, the Colleague object is confirmed that it can attack. The Mediator calls this method whenever it wants a unit to stop attacking. Programmatically we are not maintaining any reference between Colleague objects. They only communicate with the Mediator and the Mediator communicates with them.
Complete code for the above example
Direct insight into sulfiphilicity-lithiophilicity design of bifunctional heteroatom-doped graphene mediator toward ... - ScienceDirect.com
Direct insight into sulfiphilicity-lithiophilicity design of bifunctional heteroatom-doped graphene mediator toward ....
Posted: Thu, 02 Sep 2021 06:21:51 GMT [source]
The communication between the components can get rather confusing if there is a large number of components. The connection is often made in the connector of the component, where a mediator object is supplied as a parameter. Concrete Mediators contain the interactions of distinct components. Concrete mediators frequently maintain track of all the components they govern and, in some cases, manage their whole lifespan. The Mediator is an entity that governs how a group of items communicate with one another.
The sender doesn’t know who will end up handling the request, and the receiver doesn’t know who sent it in the first place. Each talks tothe Mediator, which in turn knows and conducts the orchestration ofthe others. The "many to many" mapping between colleagues that wouldotherwise exist, has been "promoted to full object status". This newabstraction provides a locus of indirection where additional leveragecan be hosted. Although the different participants on the call seem to be communicating directly, they don't.
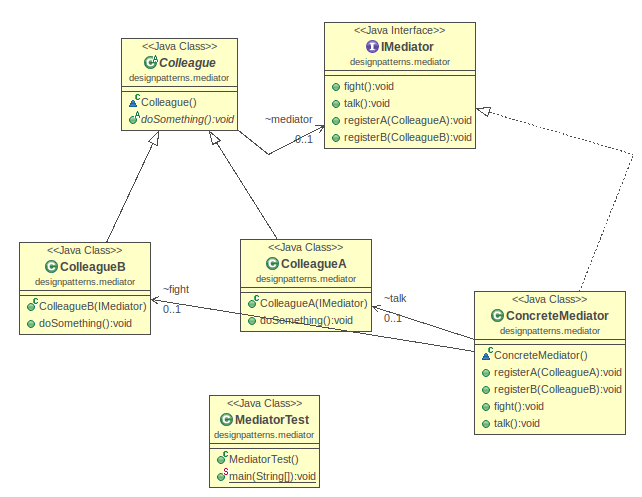
The implementation
Let’s write some test code so we can observe the Mediator Pattern in action. The participants in this design are the mediator, the concrete mediator, and one or more participant types. Note that the participant type is sometimes called a colleague.
MediatorPatternDemo, our demo class, will use User objects to show communication between them. In a smart house, there are four smart workers [Alarm, Coffeepot, Calendar, and Sprinkler]; each one of them can do its specific staff as predefined from the owner. When the time comes, the alarm informs the Coffee Machine to start brewing the coffee. But there are more complex conditions, For example, In weekends, the routine will differ. When something important occurs within or to a component, it must only notify the mediator. If the mediator receives a notification, it can easily identify the sender, which might be just enough to determine which component should be triggered.
Mediator Pattern vs. Adapter Pattern
The Mediator pattern suggests that you should cease all direct communication between the components which you want to make independent of each other. Instead, these components must collaborate indirectly, by calling a special mediator object that redirects the calls to appropriate components. As a result, the components depend only on a single mediator class instead of being coupled to dozens of their colleagues. In the programming world, you can easily model this requirement by creating classes for each armed unit. In each class, whenever its object is about to start attacking, you can implement the logic to notify objects of the other classes.
Most importantly, they don’t need to have any knowledge of one another. Thus the mediator pattern helps you write well-structured, maintainable, and easily testable code. The Mediator design pattern is a behavioral pattern that defines an object, the mediator, to centralize communication between various components or objects in a system. According to GoF definition, mediator pattern defines an object that encapsulates how a set of objects interact.
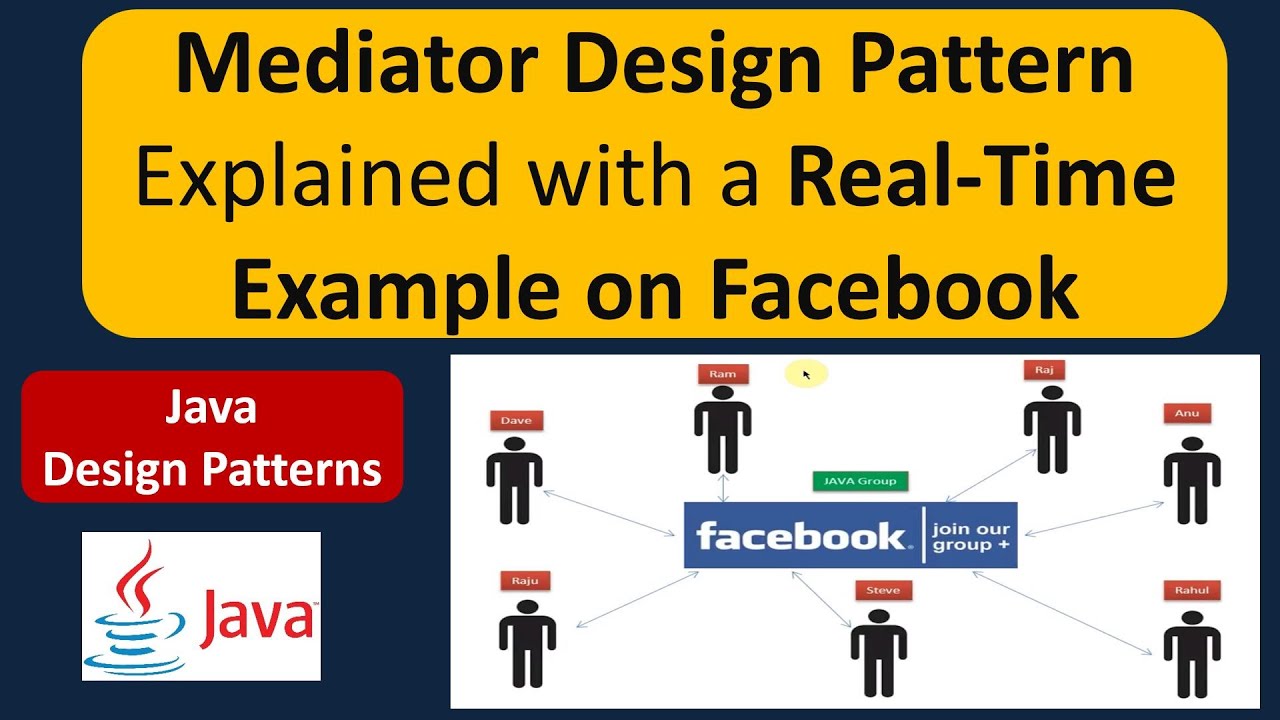
Instead of having the pilots talk to each other directly, which would probably end up being quite chaotic, the pilots talk the air traffic controller. The air traffic controller makes sure that all planes receive the information they need in order to fly safely, without hitting the other airplanes. In no way, the participants will communicate directly with each other.
This pattern helps reduce (or eliminate) coupling between modules in the system by moving that coupling into a Mediator module or class. The Mediator is responsible for coordinating and controlling the interactions of the various modules. This structural code demonstrates the Mediator pattern facilitating loosely coupled communication between different objects and object types.
So, please modify the Main method of the Program class as shown below. Here, we create the FacebookGroupMediator object, create several Facebook users, and then register all the users to the mediator object. Then, we send a message to the Mediator using the Dave and Rajesh user. The following client code is self-explained, so please go through the comment lines for a better understanding. These are the classes that communicate with each other via the mediator.
When you wish to reuse component classes in multiple situations, this interface is critical. You can link the component with a different mediator implementation as long as the component communicates with its mediator using the general interface. The Mediator interface specifies communication methods with components, which typically comprise only a single notification method. The Main method of the Program class is going to be the client.